分享4个常用C#枚举扩展方法,包括字符串转枚举和数字转枚举等,让枚举在实际应用中更加灵活
|
admin
2024年11月27日 9:18
本文热度 1665
|
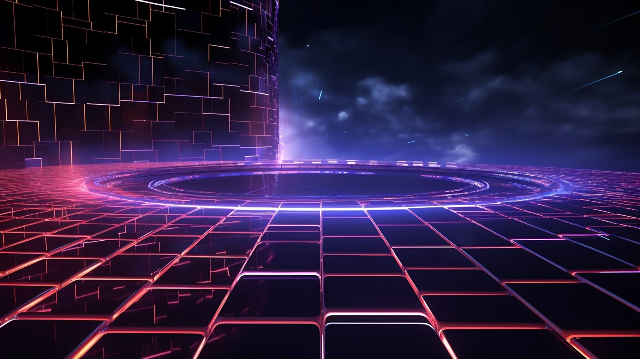
前言
嗨,大家好!
在 C# 的世界里,枚举(Enum)让我们可以用更直观的方式来表示一组相关的常量,比如 HTTP 方法、状态码等。
通过扩展方法,我们可以为枚举赋予更多的功能,使其在实际应用中更加灵活。
今天,我们要分享的就是 4 个我在工作中常用的枚举扩展方法:获取枚举描述、描述转枚举、字符串转枚举和数字转枚举等。
这些方法在我的工作中帮助挺大的,希望对你也有所启发,让我们一起来看看吧!
枚举扩展方法
以下是完整的枚举扩展方法,留意代码中的注释:
public static class EnumUtil
{
/// <summary>
/// 获取枚举描述
/// </summary>
/// <param name="value"></param>
/// <returns></returns>
public static string ToDescription(this Enum value)
{
if (value == null) return "";
System.Reflection.FieldInfo fieldInfo = value.GetType().GetField(value.ToString());
object[] attribArray = fieldInfo.GetCustomAttributes(false);
if (attribArray.Length == 0)
{
return value.ToString();
}
else
{
return (attribArray[0] as DescriptionAttribute).Description;
}
}
/// <summary>
/// 根据描述获取枚举值
/// </summary>
/// <typeparam name="T"></typeparam>
/// <param name="description"></param>
/// <returns></returns>
public static T GetEnumByDescription<T>(this string description) where T : Enum
{
if (!string.IsNullOrWhiteSpace(description))
{
System.Reflection.FieldInfo[] fields = typeof(T).GetFields();
foreach (System.Reflection.FieldInfo field in fields)
{
//获取描述属性
object[] objs = field.GetCustomAttributes(typeof(DescriptionAttribute), false);
if (objs.Length > 0 && (objs[0] as DescriptionAttribute).Description == description)
{
return (T)field.GetValue(null);
}
}
}
throw new ArgumentException(string.Format("{0} 未能找到对应的枚举.", description), "Description");
}
/// <summary>
/// 数字转枚举
/// </summary>
/// <returns></returns>
public static T ToEnum<T>(this int intValue) where T : Enum
{
return (T)Enum.ToObject(typeof(T), intValue);
}
/// <summary>
/// 字符串转枚举
/// </summary>
/// <typeparam name="T"></typeparam>
/// <param name="enumString"></param>
/// <returns></returns>
public static T ToEnum<T>(this string enumString) where T : Enum
{
return (T)Enum.Parse(typeof(T), enumString);
}
}
使用
1. 创建枚举
首先,我们来定义一个枚举,表示 HTTP 方法类型:
public enum HttpMethodType
{
[Description("查询数据")]
GET = 1,
[Description("保存数据")]
POST = 2,
[Description("更新数据")]
PUT = 3,
[Description("删除数据")]
DELETE = 4
}
2. 使用扩展方法
接下来,我们在 Program.cs
文件里使用这些扩展方法:
using EumnSample;
// 1. 获取枚举的描述
HttpMethodType httpMethodType = HttpMethodType.GET;
string getMethodDesc = httpMethodType.ToDescription();
Console.WriteLine(getMethodDesc);
// 2. 根据描述获取相应的枚举
string postMethodDesc = "保存数据";
HttpMethodType postMethodType = postMethodDesc.GetEnumByDescription<HttpMethodType>();
Console.WriteLine(postMethodType.ToString());
// 3. 根据枚举名称获取相应的枚举
string putMethodStr = "PUT";
HttpMethodType putMethodType = putMethodStr.ToEnum<HttpMethodType>();
Console.WriteLine(putMethodType.ToString());
// 4. 根据枚举的值获取相应的枚举
int delMethodValue = 4;
HttpMethodType delMethodType = delMethodValue.ToEnum<HttpMethodType>();
Console.WriteLine(delMethodType.ToString());
3. 运行
运行程序,即可看到枚举扩展方法的强大功能,如图所示:
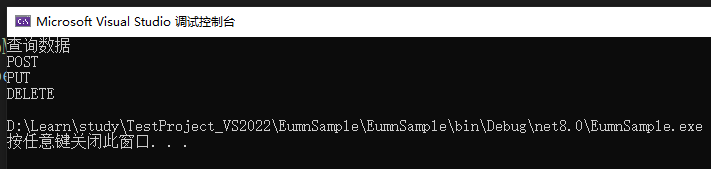
总结
通过这几个枚举扩展方法,我们可以更加灵活地操作枚举,让代码更加优雅和高效。
该文章在 2024/11/27 9:18:52 编辑过